主题
今天的主题是使用springboot starter
和阿里云的AI识别sdk构建一个身份证识别应用。
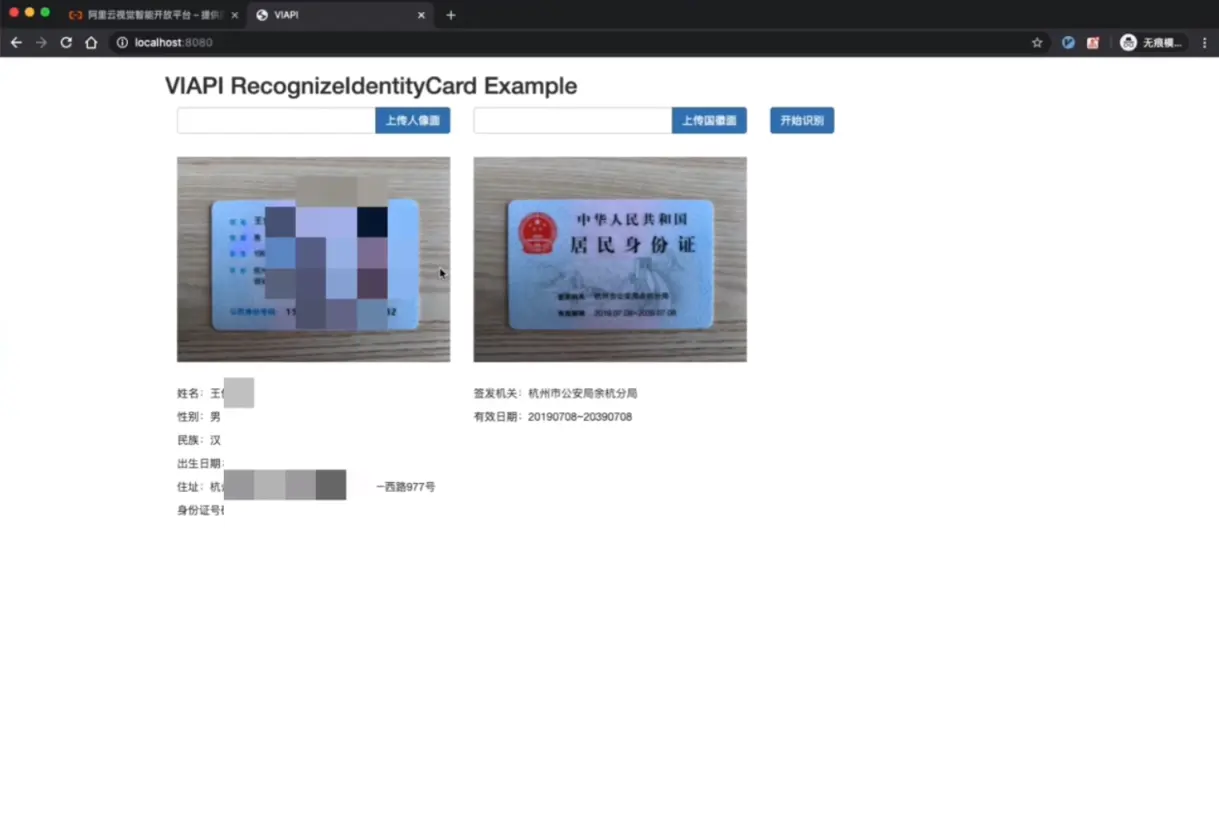
主要依赖项
主要逻辑
用户在web端上传身份证的正反面的照片,然后传递到springboot
,然后由后端将图像传递到阿里云的识别服务,等待识别服务响应识别结果,最后将结果渲染到thymeleaf
模板中返还给用户实现完整逻辑。
代码实现分析解读
Controller层上传文件部分
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| @PostMapping("/upload") public String uploadFile(@RequestParam("face") MultipartFile face, @RequestParam("back") MultipartFile back, RedirectAttributes attributes) { if (face.isEmpty() || back.isEmpty()) { attributes.addFlashAttribute("message", "Please select a file to upload."); return "redirect:/"; } String errorMessage = null; try { Path dir = Paths.get(uploadDirectory); if (!Files.exists(dir)) { Files.createDirectories(dir); } if (!face.isEmpty()) { String filename = saveFile(face); Map<String, String> res = ocrService.RecognizeIdCard(uploadDirectory + filename, "face"); faceImages.add("/images/" + filename); faceResults.add(res); } if (!back.isEmpty()) { String filename = saveFile(back); Map<String, String> res = ocrService.RecognizeIdCard(uploadDirectory + filename, "back"); backImages.add("/images/" + filename); backResults.add(res); } } catch (TeaException e) { e.printStackTrace(); errorMessage = JSON.toJSONString(e.getData()); } catch (Exception e) { e.printStackTrace(); errorMessage = e.getMessage(); } if (StringUtils.isNotBlank(errorMessage)) { attributes.addFlashAttribute("message", errorMessage); } return "redirect:/"; }
|
OCR服务
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
public Map<String, String> RecognizeIdCard(String filePath, String side) throws Exception { RecognizeIdentityCardAdvanceRequest request = new RecognizeIdentityCardAdvanceRequest(); request.imageURLObject = Files.newInputStream(Paths.get(filePath)); request.side = side; RecognizeIdentityCardResponse response = ocrClient.recognizeIdentityCardAdvance(request, runtime);
if ("face".equals(side)) { return JSON.parseObject(JSON.toJSONString(response.data.frontResult), new TypeReference<Map<String, String>>() {}); } else { return JSON.parseObject(JSON.toJSONString(response.data.backResult), new TypeReference<Map<String, String>>() {}); } }
|